How to Display Yes or No Instead of Checkbox while binding Boolean value with GridView
How to Display “Yes” or “No” Instead of Checkbox while binding Boolean value with GridView ?
Let’s consider you have a simple class “Student” with some student records.
[sourcecode language="csharp"]
/// <summary>
/// Student Class
/// </summary>
public class Student
{
public int Roll { get; set; }
public string Name { get; set; }
public bool Status { get; set; }
}
/// <summary>
/// Handles the Load event of the Page control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="System.EventArgs"/> instance containing the event data.</param>
protected void Page_Load(object sender, EventArgs e)
{
List<Student> students = new List<Student>();
students.Add(new Student { Roll = 1, Name = "Abhijit", Status=true});
students.Add(new Student {Roll=2,Name="Manish",Status=false});
students.Add(new Student { Roll = 3, Name = "Atul", Status = true });
GridView2.DataSource = students;
GridView2.DataBind();
}
[/sourcecode]
Now if you run the application you will get below out put Which is the default behavior of GridView
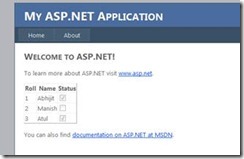
Now Change the binding during on RowDataBound of GridView
[sourcecode language="csharp"]
/// <summary>
/// Handles the RowDataBound event of the GridView2 control.
/// </summary>
/// <param name="sender">The source of the event.</param>
/// <param name="e">The <see cref="System.Web.UI.WebControls.GridViewRowEventArgs"/> instance containing the event data.</param>
protected void GridView2_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
Student s = (Student)e.Row.DataItem;
if (s.Status == true)
{
e.Row.Cells[2].Text = "1";
}
else
{
e.Row.Cells[2].Text = "0";
}
}
}
[/sourcecode]
Now if you run the application, your output will be looks like below.
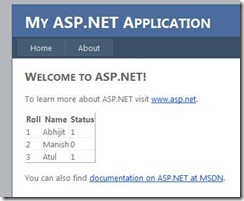
Instead of 1/0 you can use “Yes/No” or whatever value you want instead of Checkbox.

Sagar S Bhanushali
Comments
Post a Comment